Important Dot Net Core Interview Questions 2023
In this article, we will mostly be asked Dot net core interview questions and answers. Before that let’s understand some background about ASP.NET Core.
ASP.NET Core is a free, open-source, and cloud-optimized web framework that can run on Windows, Linux, or Mac. You can say that it is the new version of ASP.NET.
ASP.NET Core is designed to be deployed on the cloud as well as on-premises. Developers can now build cloud-based web applications, IoT (Internet of Things), and mobile backend applications using the ASP.NET Core framework which can run on Windows, Linux, and Mac operating systems.
You can check out the article on introduction to asp.net core to understand them in more detail. Let’s start with Dot net core interview questions.
Q 1. What is the ASP.NET Core
- ASP.NET Core is a free, open-source and cloud optimized web framework which can run on Windows, Linux, or Mac.
- The framework is a complete rewrite from scratch in order to make it open source, modular and cross-platform
- It was initially launched as ASP.NET 5 but then it was renamed to ASP.NET Core
Q 2. Why do we need an ASP.NET Core or features of ASP.NET Core
- Supports Multiple Platforms: ASP.NET Core applications can run on Windows, Linux, and Mac. So you don’t need to build different apps for different platforms using different frameworks.
- Fast and lightweight: ASP.NET Core no longer depends on System.Web.dll for browser-server communication. ASP.NET Core allows us to include packages which we need for our application. This reduces the request pipeline and improves the performance and scalability.
- IoC Container: It includes built-in IoC container for automatic dependency injection which makes it maintainable and testable. Integration with Modern UI Frameworks.
- Hosting: ASP.NET Core web application can be hosted on multiple platforms with any web server such as IIS, Apache etc. It is not dependent only on IIS as a standard .NET Framework.
- Code Sharing: It allows you to build a class library which can be used with other .NET frameworks such as .NET Framework 4.x or Mono. Thus a single code based can be shared across frameworks.
Q 3. What is .NET Core
Many people are confused between ASP.NET Core and .NET Core. Please note that ASP.NET Core and .NET Core are not the same. They are different, just like ASP.NET and .NET Framework are different.
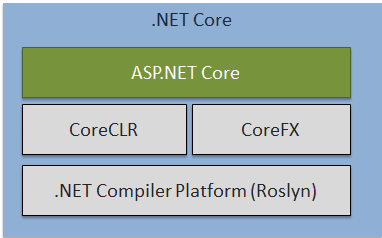
- .NET Core is a fast, lightweight, modular and open source framework for creating web applications and services that run on Windows, Linux and Mac.
- It is a platform on which ASP.NET Core application runs.
- .NET Core is named “Core” because it includes core features of the .NET framework.
- The main objective of .NET Core is to make .NET framework open source, and cross-platform compatible so that it can be used in resource-constrained environments.
Q 4. What is the startup class in ASP.NET core
- It is like Global.asax in the traditional .NET application. As the name suggests, it is executed first when the application starts.
- Startup class is the entry point of the ASP.NET Core application. Every .NET Core application must have this class.
- It is used to configures the services which are required by the app.
- It is used to defines the app's request handling pipeline as a series of middleware components.
- Multiple Startup classes can also be defined for different environments
- It is not necessary that class name must "Startup", it can be anything, we can configure startup class in Program class.
Q 5. What is the use of the ConfigureServices method
- This is an optional method of startup class. It can be used to configure the services that are used by the application
- The ConfigureServices method is called before Configure method.
- This method calls first when the application is requested for the first time.
- Using this method, we can add the services to the DI container, so services are available as a dependency in controller constructor.
Q 6. What is the use of the Configure method
- This method is used to add middleware components to the IApplicationBuilder instance that's available in Configure method.
- This method is used to specify how the ASP.NET application will respond to individual HTTP requests.
- It accepts IApplicationBuilder as a parameter and also it has two optional parameters: IHostingEnvironment and ILoggerFactory.
- Using this method, we can configure built-in middleware such as routing, authentication, session, etc. as well as third-party middleware.
Q 7. What is middleware
- In ASP.NET Core, Middleware is a piece of code that are assembled into an application pipeline to handle an HTTP request or response.
- Middleware component has access to both the incoming request and the outgoing response.
- A Middleware component may handle the request and decide not to call the next middleware in the pipeline. This is called short-circuiting the request pipeline.
- Middleware component are must be executed in order they added in pipeline. So need take care of sequence execution of the middle otherwise it will not function as expected
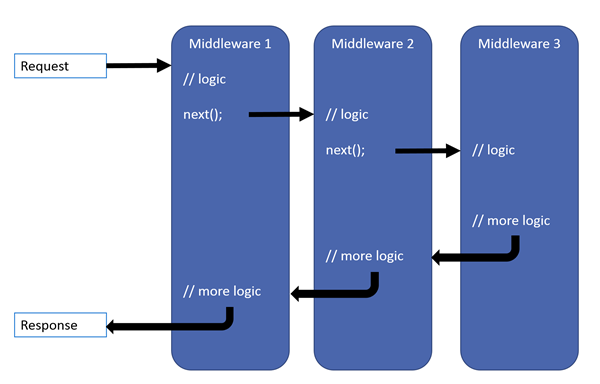
Q 8. Difference between IApplicationBuilder.Use() and IApplicationBuilder.Run()
- We can use both the methods in Configure methods of startup class. Both are used to add middleware delegate to the application request pipeline.
- The middleware adds using IApplicationBuilder.Use may call the next middleware in the pipeline whereas the middleware adds using IApplicationBuilder.Run method never calls the subsequent ore next middleware.
- To configure multiple middleware, use Use() extension method. It is similar to Run() method except that it includes next parameter to invoke next middleware in the sequence.
Q 9. What is routing in ASP.NET Core and types of routing
Routing in ASP.NET Core is the process of mapping incoming requests to application logic that are resides in controllers and methods.
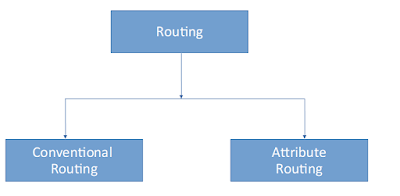
- Conventional routing: The route is determined based on conventions that are defined in route templates, at run time, it will will map requests to the controllers and actions or methods.
- Attribute-based routing: The route is determined based on attributes that you set on your controllers and methods.
Q 10. What are the JSON files available in ASP.NET Core
- global.json: it is use to define solution level settings in global.json file
- launchsettings.json: it is used to define project specific settings associated with each profile Visual Studio is configured to launch the application, including any environment variables that should be used. You can define framework for your project for compliation and debugging for specific profiles.
- appsettings.json: it is used to store custom application setting, DB connection strings,Logging etc
- bundleconfig.json: can define the configuration for bundling and minification for the project.
- project.json: it is used for storing all project level configuration settings
- bower.json: Bower is a package manager for the web. Bower manages components that contain HTML, CSS, JavaScript, fonts or even image files. Bower installs the right versions of the packages you need and their dependencies
Q 11. What is dependency injection
Dependency Injection (DI): its a software design pattern that allows us to develop loosely coupled code. Dependency Injection is a great way to reduce tight coupling between software components. Dependency Injection also enables us to better manage future changesand other complexity in our software. The purpose of DI is to make code maintainable.
Tightly Coupling: The software components were dependent on each other in such a way that, for a small change in one component, you might need to change a lot in the dependent component. This is not good practice in software development. Simplest way to understand is when we get an instance by using a new keyword that class or service is tightly coupled.
Loosely Coupling: In this case, the software components slightly depend on each other. If we need to change one component then it will not affect much more on the dependent component. We can achieve this situation through interfaces. The classes can communicate with each other with the help of an interface. Service Lifetime
Q 12. What are the service lifetime
it is important to understand what is service lifetime. When we are registering our service then we need to register with the correct lifetime to define lifetime service issues or shares. The Built-in IoC container manages the lifetime of a registered service type. It automatically disposes a service instance based on the specified lifetime.
In asp.net core There are 3 options for this with the built-in DI container in ASP.NET Core:
- Singleton: It means only a single instance will ever be created. That instance is shared between all components that require it. The same instance is thus always used.
- Scoped: means an instance is created once per scope. A scope is created on every request to the application, thus any components registered as Scoped will be created once per request.
- Transient: instance are created every time they are requested and are never shared.
Q 13. Can ASP.NET Core work with the .NET framework?
Yes. ASP.NET core application works with full .NET framework via the .NET standard library. We need to make use of .NET standard library make both application compatible with each other.
Q 14. Why do we need a program.cs file
In an ASP.NET Core project we have a file with name Program.cs. In this file we have asp.net core main method as public static void Main() method
The most important point that you need to keep in mind is, the ASP.NET Core Web Application initially starts as a Console Application and the Main() method is the entry point to the application. So, when we execute the ASP.NET Core Web application, first it looks for the Main() method and this is the method from where the execution starts. The Main() method then configures ASP.NET Core and starts it. At this point, the application becomes an ASP.NET Core web application.
Q 15. What is Kestrel
Kestrel is a cross-platform web server built for ASP.NET Core. It is based on asynchronous I/O library called “libuv” primarily developed for Node.js It is default web server, but you still can use IIS, Apache, Nginx etc.
Usually, it is used as an edge-server, which means it is the server which faces the internet and handles HTTP web requests from clients directly. It is a listening server with a command-line interface.
Advantages of Kestrel:
- Lightweight and fast.
- Cross-platform and supports all versions of .NET Core.
- Supports HTTPS
- Easy configuration
Q 16. What is metapackages in .net cor
The framework .NET Core 2.0 introduced Metapackage that includes all the supported package by ASP.NET code with their dependencies into one package. It helps us to do fast development as we don’t require to include the individual ASP.NET Core packages. The assembly Microsoft.AspNetCore.All is a meta package provide by ASP.NET core.
Q 17. What is wwwroot folder in ASP.NET Core
In ASP.NET Core, all the static resources, such as CSS, JavaScript, and image files are kept under the wwwroot
folder (default).
Static files are typically located in the web root (wwwroot) folder and its default place where we can serve up files directly from the file system when we create your asp.net core application
Q 18. How to enable Session in ASP.NET Core
- The middleware for the session is provided by the package Microsoft.AspNetCore.Session.
- To use the session in ASP.NET Core application, we need to add this package to csproj file and add the Session middleware to ASP.NET Core request pipeline
public void ConfigureServices(IServiceCollection services)
{
services.AddSession(options => {
options.IdleTimeout = TimeSpan.FromMinutes(1);//You can set Time
});
services.AddMvc();
}
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
app.UseStaticFiles();
app.UseSession();
app.UseMvc(routes =>
{
routes.MapRoute(
name: "default",
template: "{controller=Home}/{action=Index}/{id?}");
});
}
Q 19. What is In-memory cache
With ASP.NET Core , it is now possible to cache the data within the application. In-memory cache is the simplest way of caching by ASP.NET Core that stores the data in memory on web server. This is known as In-Memory Caching in ASP.NET Core. It is a service that you can incorporate into your application using dependency injection. IMemoryCache interface instance in the constructor enables the In-memory caching service via ASP.NET Core dependency Injection .
Apps running on multiple server should ensure that sessions are sticky if they are using in-memory cache. Sticky Sessions responsible to redirect subsequent client requests to same server.
Q 20. How to enable the in-memory cache in ASP.NET Core project
You can enable the in-memory cache in the ConfigureServices method in the Startup class.
public void ConfigureServices(IServiceCollection services)
{
services.AddMvc();
services.AddMemoryCache();
}
Please check below video version of this tutotial.