ASP.NET CORE Mvc Controllers
In this tutorial will discuss what is a controller in asp.net core mvc and role of controller in asp.net core mvc The Controllers in ASP.NET Core MVC application are the classes and its derived from the base class System.Web.Mvc.Controller
Controller class having a variety of public methods. These public methods are called as actions (action methods) in the Controller class.
These action methods are the methods of a Controller which is actually going to handle the incoming HTTP Requests.
The controller class name must be suffixed with word “Controller”. For example HomeController, StudentController. If you have not gave suffix then it will throw error of resource not found.
A controller is used to define and group a set of actions. An action (or action method) is a method on a controller which handles requests. Controllers logically group similar actions together. This aggregation of actions allows common sets of rules, such as routing, caching, and authorization, to be applied collectively. Requests are mapped to actions through routing.
Any request come to our web application from the browser. the controller in the MVC architecture that handles the incoming http request and identify the accurate model, sends response back to the user action.
Once done with adding controller we need to resolve the dependency of ISudentRepository into the Stratup.cs in ConfigureServices method
public class Startup { // This method gets called by the runtime. Use this method to add services to the container. // For more information on how to configure your application, visit https://go.microsoft.com/fwlink/?LinkID=398940 public void ConfigureServices(IServiceCollection services) { services.AddMvc(); services.AddScoped<IStudentRespository, StudentRespository>(); } // This method gets called by the runtime. Use this method to configure the HTTP request pipeline. public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } app.UseRouting(); app.UseEndpoints(endpoints => { endpoints.MapControllerRoute( name: "default", pattern: "{controller=Home}/{action=Index}/{id?}"); }); } }
Return JSON Data:
We need to use JsonResult return type to get JSON data from our GetStudent action method. This method always going to returns the data in JSON format irrespective of the content negotiation of respsone type the method
/// GTechFluent Student Controller ///
public class StudentController : Controller {
protected readonly IStudentRespository _studentRespository;
public StudentController(IStudentRespository studentRespository)
{
_studentRespository = studentRespository;
}
public JsonResult GetStudent(int id)
{
Student student = _studentRespository.GetStudent(id);
return Json(student);
}
}
Return Content Data:
/// GTechFluent Student Controller ///
public class StudentController : Controller {
protected readonly IStudentRespository _studentRespository;
public StudentController(IStudentRespository studentRespository)
{
_studentRespository = studentRespository;
}
public ContentResult GetStudent(int id)
{
Student student = _studentRespository.GetStudent(id);
string result = JsonConvert.SerializeObject(student);
return Content(result);
}
}
Return View:
/// GTechFluent Student Controller ///
public class StudentController : Controller {
protected readonly IStudentRespository _studentRespository;
public StudentController(IStudentRespository studentRespository)
{
_studentRespository = studentRespository;
}
public IActionResult GetStudent(int id)
{
Student student = _studentRespository.GetStudent(id);
return View(student);
}
}
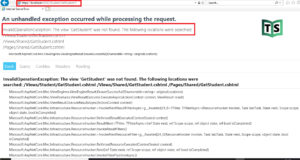
You can watch our video version of this tutorial with step by step explanation.