ASP.NET CORE Mvc Views
In this tutorial will discuss on views in asp.net core mvc and understand different ways of returning view.
Views in MVC
- A view is an HTML template with embedded Razor markup which use to represent data.
- A view is used to display data using the model class object.
- A view file has .cshtml extension as we using the programming language is C# in our ASP.NET Core MVC application
View Files Location.
- In MVC view files are located in the Views folder.
- View files of the specific controller are stored under a sub-folder in the Views folder and that sub-folder has the same name as the controller.
In our application, We have two controllers HomeController and StudentController. We have one action on each controller as of now. We will be modifying going forward as per requirement,
The HomeController has below action method.
- Index()
The StudentController is created with the following four action methods.
- Details()
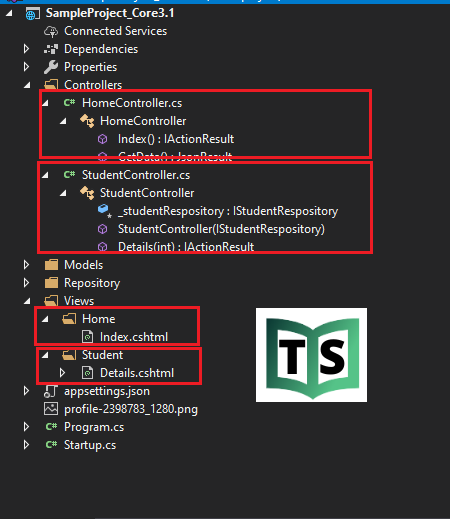
There are separate folder is created for each controller within the Views Folder by MVC. The Home Controller is represented by a Home view folder and the Student Controller is represented by a Student view folder.
Before understanding view let make changes in action method our GetStudent to Details in StudentController and return type as ViewResult to make things clear.
Understanding View Implementation:
Our StudentController have only one action method Details and the return type of the Details() action method is ViewResult, so this action method is going to return a view. In order to return a view, here we are using the View() extension method which is provided by Controller Base class. When run our application and navigate to the “/Student/Details/1” URL and you will see the following error. This is because we have not added view yet for Details action
InvalidOperationException: The view ‘Details’ was not found. The following locations were searched: /Views/Student/Details.cshtml
/Views/Shared/Details.cshtml
/Pages/Shared/Details.cshtml
MVC looks for this file in the following 3 locations in the order specified
- First in “/Views/Home/” folder because the Controller name is StudentController
- Then in “/Views/Shared/” folder
- Finally in “/Pages/Shared/” folder
Adding View:
We need to create the Views folder at the root level of the application. After creating the Views Folder, then create a Student folder within that Views folder.
Now Right-click on the Student folder and select Add => View
Provide the name as Details.cshtml and select the Template after that select model class Student.cs as we we need to display student details and click on the Add button to finish.
[NonAction] public virtual ViewResult View(); [NonAction] public virtual ViewResult View(string viewName); [NonAction] public virtual ViewResult View(string viewName, object model); [NonAction] public virtual ViewResult View(object model);
/// /// GTechFluent Student Controller ///
public class StudentController : Controller { protected readonly IStudentRespository _studentRespository; public StudentController(IStudentRespository studentRespository) { _studentRespository = studentRespository; } public ViewResult Details(int id) { return View(); } }
/// /// GTechFluent Student Controller ///
public class StudentController : Controller { protected readonly IStudentRespository _studentRespository; public StudentController(IStudentRespository studentRespository) { _studentRespository = studentRespository; } public ViewResult Details(int id) { Student student = _studentRespository.GetStudent(id); return View(student); } }
/// /// GTechFluent Student Controller ///
public class StudentController : Controller { protected readonly IStudentRespository _studentRespository; public StudentController(IStudentRespository studentRespository) { _studentRespository = studentRespository; } public ViewResult Details(int id) { return View(“StudentInfo”); } }
/// /// GTechFluent Student Controller ///
public class StudentController : Controller { protected readonly IStudentRespository _studentRespository; public StudentController(IStudentRespository studentRespository) { _studentRespository = studentRespository; } public ViewResult Details(int id) { Student student = _studentRespository.GetStudent(id); return View(“StudentInfo”,student); } }
In our next tutorial will discuss different ways of passing the data between controller to view.
You can watch our video version of this tutorial with step by step explanation.