ASP.NET CORE Middleware
In this tutorials will discuss and understand what is the middleware and significant of the middleware’s.
What is Middleware ASP. NET Core?
Middleware Pipeline
The ASP.NET core request pipeline consists a sequence of request delegates, called one after the next, as this diagram shows (the thread of execution follows the black arrows):
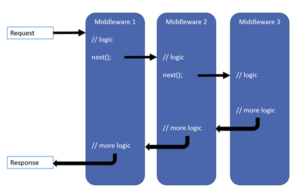
Each delegate has the opportunity to perform operations before and after the next delegate. Any delegate can choose to stop passing the request on to the next delegate, and instead handle the request itself.
You can see an example of setting up the request pipeline in the default web site template.
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
app.UseHttpsRedirection();
app.UseMiddleWareBefore();
app.UseRouting();
app.UseMiddleWareAfter();
app.UseAuthorization();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllers();
});
}
Built in Middleware in ASP. NET Core
Exception Handling
- UseDeveloperExceptionPage() & UseDatabaseErrorPage(): used in development to catch run-time exceptions
- UseExceptionHandler(): used in production for run-time exceptions
Calling these methods first ensures that exceptions are caught in any of the middleware components that follow. For more information, check out the detailed post on Handling Errors in ASP .NET Core, earlier in this series.
HSTS & HTTPS Redirection:
- UseHsts(): used in production to enable HSTS (HTTP Strict Transport Security Protocol) and enforce HTTPS.
- UseHttpsRedirection(): forces HTTP calls to automatically redirect to equivalent HTTPS addresses.
Calling these methods next ensure that HTTPS can be enforced before resources are served from a web browser. For more information, check out the detailed post on Protocols in ASP .NET Core: HTTPS and HTTP/2.
Static Files:
- UseStaticFiles(): used to enable static files, such as HTML, JavaScript, CSS and graphics files. Called early on to avoid the need for authentication, session or MVC middleware.
Calling this before authentication ensures that static files can be served quickly without unnecessarily triggering authentication middleware. For more information, check out the detailed post on JavaScript, CSS, HTML & Other Static Files in ASP .NET Core.
Cookie Policy:
- UseCookiePolicy(): used to enforce cookie policy and display GDPR-friendly messaging
Calling this before the next set of middleware ensures that the calls that follow can make use of cookies if consented. For more information, check out the detailed post on Cookies and Consent in ASP .NET Core.
Authentication, Authorization & Sessions:
- UseAuthentication(): used to enable authentication and then subsequently allow authorization.
- UseSession(): manually added to the Startup file to enable the Session middleware.
Calling these after cookie authentication (but before the endpoint routing middleware) ensures that cookies can be issued as necessary and that the user can be authenticated before the endpoint routing kicks in.
Endpoint Routing:
- UseEndpoints(): usage varies based on project templates used for MVC, Razor Pages and Blazor.
- endpoints.MapControllerRoute(): set the default route and any custom routes when using MVC.
- endpoints.MapRazorPages(): sets up default Razor Pages routing behavior
- endpoints.BlazorHub(): sets up Blazor Hub
You can watch our video version of this tutorials with step by step explanation