ASP.NET CORE Serving Static Files
In this tutorial will discuss how to get static files which require for our application like CSS, html, JS and images.
Static files are required for your application such as JavaScript files, CSS files, html files and images or icons.
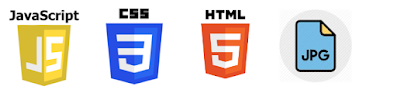
Serving Static Files
Static files are typically located in the web root (wwwroot) folder and its default place where we can serve up files directly from the file system when we create your asp.net core application
Static files can be stored in any folder under the web root and accessed with a relative path to that root. For example, when you create a default Web application project using Visual Studio, there are several folders created within the wwwroot folder – css, images, lib and js.
When you run your application and try to browse https://localhost:44325/index.html into the browser, you will get file not found exception thrown.
This because there is no piece of middleware configure to serve any file on the file system. In order for static files to be served, you must configure the Middleware to add static files to the pipeline. The static file middleware can be configured by adding a dependency on the Microsoft.AspNetCore.StaticFiles package to your project and then calling the UseStaticFiles extension method from Startup.Configure:
public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } app.UseHttpsRedirection(); app.UseStaticFiles(); app.UseCors(c => c.AllowAnyOrigin()); app.UseRouting(); app.UseEndpoints(endpoints => { endpoints.MapControllers(); }); }
If the static file finds a file into to the file system, so it will serve up that file and not call the next piece of middleware.
If it doesn’t find a matching file, then it will simply continue with the next piece of middleware.
After making above changes and run our application access index.html file.
Now suppose you are converting your existing mvc application to asp.net core application and in this case you need to move your folder inside the wwwroot but in some cases you want it from the existing folder without moving to wwwroot. Achieve we need to use StaticFileOptions as below.
- Let create folder on application with MyStaticFiles
- Add new html file test.html inside MyStaticFiles folder
- Make Below changes in your startup.cs class file
- Run application and access https://localhost:44325/staticfiles/test.html into the browser.
public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } app.UseHttpsRedirection() app.UseStaticFiles(); app.UseStaticFiles(new StaticFileOptions() { FileProvider = new PhysicalFileProvider( Path.Combine(Directory.GetCurrentDirectory(), @"MyStaticFiles")), RequestPath = new PathString("/StaticFiles") }) app.UseCors(c => c.AllowAnyOrigin()); app.UseRouting(); app.UseEndpoints(endpoints => { endpoints.MapControllers(); }); }
Default page
Now if you want index.html to be your default file, this is a feature that IIS has always had. Means whenever an application starts we need some static page to be loaded. To Enable default page application we need to configure UseDefaultFiles middleware to configure. This middleware looks for 4 types of default pages.
- index.htm
- index.html
- default.htm
- default.html
public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } app.UseHttpsRedirection(); app.UseDefaultFiles(); app.UseStaticFiles(); app.UseStaticFiles(new StaticFileOptions() { FileProvider = new PhysicalFileProvider( Path.Combine(Directory.GetCurrentDirectory(), @"MyStaticFiles")), RequestPath = new PathString("/StaticFiles") }) app.UseCors(c => c.AllowAnyOrigin()); app.UseRouting(); app.UseEndpoints(endpoints => { endpoints.MapControllers(); }); }
You can watch our video version of this tutorial with step by step explanation.